Overview of VHDL
VHDL was developed in the early 1980s by the U.S. Department of Defense as a standard language for describing digital systems. It was later adopted by the Institute of Electrical and Electronics Engineers (IEEE) as IEEE Standard 1076. Since then, VHDL has become one of the most widely used hardware description languages in the electronics industry.
Key features of VHDL
-
Strong typing: VHDL is a strongly typed language, which means that every object must be declared with a specific type before it can be used. This helps catch errors at compile time and makes the code more readable and maintainable.
-
Concurrency: VHDL supports concurrent statements, allowing multiple processes to execute simultaneously. This is essential for modeling the parallel nature of digital circuits.
-
Hierarchical design: VHDL allows for hierarchical design, where a complex system can be broken down into smaller, more manageable subsystems. This makes the design process more organized and easier to understand.
-
Extensibility: VHDL provides a mechanism for extending the language through user-defined types, functions, and procedures. This allows designers to create reusable components and libraries.
VHDL Design Flow
The typical VHDL design flow consists of the following steps:
-
Design specification: The desired functionality and requirements of the digital system are defined.
-
VHDL coding: The system is described using VHDL code, which includes the entity (interface) and architecture (implementation) of each component.
-
Simulation: The VHDL code is simulated using a simulator to verify its functionality and detect any errors.
-
Synthesis: The VHDL code is synthesized into a gate-level netlist, which represents the physical implementation of the design.
-
Place and route: The gate-level netlist is mapped onto a specific hardware platform, such as an FPGA or ASIC, and the physical layout is generated.
-
Verification: The final design is verified through post-layout simulation and physical testing to ensure it meets the original specifications.
VHDL Language Elements
VHDL consists of several key language elements that are used to describe digital systems:
Entities and Architectures
An entity defines the interface of a component, specifying its input and output ports. The architecture describes the behavior or implementation of the entity. A single entity can have multiple architectures, allowing for different implementations of the same interface.
entity myEntity is
port (
a, b : in std_logic;
c : out std_logic
);
end myEntity;
architecture myArch of myEntity is
begin
c <= a and b;
end myArch;
Signals and Variables
Signals and variables are used to store and propagate data within a VHDL design. Signals represent wires or connections between components and have a specific data type, such as std_logic
or integer
. Variables are used for temporary storage within a process and have a local scope.
signal mySignal : std_logic;
variable myVariable : integer;
Processes and Sequential Statements
Processes are the basic building blocks of VHDL architectures. They contain sequential statements that are executed in a specific order. Processes are triggered by a sensitivity list, which specifies the signals that cause the process to execute when their values change.
myProcess : process (a, b)
begin
if a = '1' and b = '1' then
c <= '1';
else
c <= '0';
end if;
end process;
Concurrent Statements
Concurrent statements are used to describe the behavior of a digital system that occurs simultaneously. They include signal assignments, component instantiations, and generate statements.
c <= a and b;
myComponent : myEntity port map (a => a, b => b, c => c);
Packages and Libraries
Packages are used to group related declarations, such as types, constants, functions, and procedures. They promote code reuse and maintainability. Libraries are collections of packages that can be shared among multiple designs.
library IEEE;
use IEEE.std_logic_1164.all;
package myPackage is
constant MY_CONST : integer := 42;
function myFunction(a : std_logic) return std_logic;
end package;
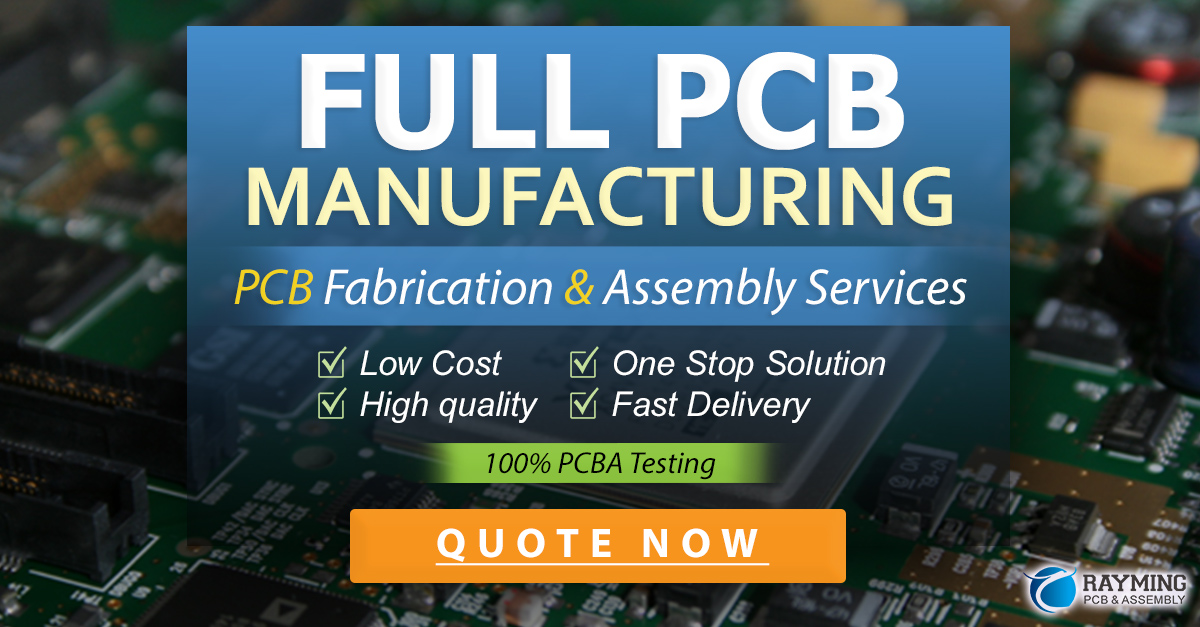
VHDL Data Types
VHDL provides a rich set of data types for describing digital systems:
Data Type | Description |
---|---|
std_logic |
A single-bit value that can take on one of nine possible values: 'U' , 'X' , '0' , '1' , 'Z' , 'W' , 'L' , 'H' , and '-' . |
std_logic_vector |
An array of std_logic elements, used for modeling buses and multi-bit signals. |
integer |
A signed integer type, typically 32 bits wide. |
natural |
An unsigned integer type, used for indexing arrays and loops. |
boolean |
A two-valued type representing true and false. |
real |
A floating-point type used for modeling analog signals and mathematical computations. |
VHDL Operators
VHDL supports a wide range of operators for performing arithmetic, logical, and relational operations on data:
Operator | Description |
---|---|
+ , - , * , / , mod , rem |
Arithmetic operators for addition, subtraction, multiplication, division, modulo, and remainder. |
and , or , nand , nor , xor , xnor , not |
Logical operators for performing bitwise and logical operations. |
= , /= , < , <= , > , >= |
Relational operators for comparing values and returning boolean results. |
& |
Concatenation operator for joining signals and vectors. |
sll , srl , sla , sra , rol , ror |
Shift and rotate operators for manipulating bits within a signal or vector. |
VHDL Simulation
Simulation is a crucial step in the VHDL design flow, allowing designers to verify the functionality of their code before synthesis and implementation. A VHDL simulator, such as ModelSim or GHDL, executes the VHDL code and displays the results in the form of waveforms or text output.
To simulate a VHDL design, a testbench is created. A testbench is a separate VHDL entity that instantiates the design under test (DUT) and provides stimulus to its inputs. The testbench also checks the outputs of the DUT against expected values to verify its correctness.
entity tb_myEntity is
end tb_myEntity;
architecture sim of tb_myEntity is
component myEntity is
port (
a, b : in std_logic;
c : out std_logic
);
end component;
signal a_tb, b_tb : std_logic;
signal c_tb : std_logic;
begin
DUT : myEntity port map (a => a_tb, b => b_tb, c => c_tb);
stim_proc : process
begin
a_tb <= '0';
b_tb <= '0';
wait for 10 ns;
assert c_tb = '0' report "Test failed for input combination 00" severity error;
a_tb <= '0';
b_tb <= '1';
wait for 10 ns;
assert c_tb = '0' report "Test failed for input combination 01" severity error;
a_tb <= '1';
b_tb <= '0';
wait for 10 ns;
assert c_tb = '0' report "Test failed for input combination 10" severity error;
a_tb <= '1';
b_tb <= '1';
wait for 10 ns;
assert c_tb = '1' report "Test failed for input combination 11" severity error;
report "All tests passed." severity note;
wait;
end process;
end sim;
VHDL Synthesis
After a VHDL design has been simulated and verified, it can be synthesized into a gate-level netlist. Synthesis is the process of transforming the VHDL code into a hardware implementation, typically targeting an FPGA or ASIC.
Synthesis tools, such as Xilinx ISE or Intel Quartus, analyze the VHDL code and optimize it for the target hardware. They perform tasks such as:
- Inferring combinational and sequential logic from the VHDL code
- Optimizing the logic for area, speed, or power consumption
- Mapping the optimized logic onto the available resources of the target device
- Generating a gate-level netlist that represents the physical implementation of the design
The synthesized netlist can then be used for place and route, where the physical layout of the design is generated and the timing constraints are verified.
VHDL Best Practices
To create efficient, maintainable, and reusable VHDL code, designers should follow these best practices:
-
Use meaningful names: Choose descriptive names for entities, architectures, signals, and variables to make the code more readable and self-documenting.
-
Indent and comment code: Use consistent indentation and comment your code to improve readability and make it easier for others (and yourself) to understand.
-
Use packages and libraries: Organize related declarations into packages and use libraries to promote code reuse and maintainability.
-
Follow a consistent coding style: Adopt a consistent coding style, including naming conventions, signal and variable declarations, and process structures.
-
Simulate early and often: Regularly simulate your VHDL code to catch errors early in the design process and ensure the functionality matches the specifications.
-
Optimize for synthesis: Write VHDL code that is optimized for synthesis, avoiding constructs that may not be efficiently implemented in hardware.
-
Use generics and parameters: Utilize generics and parameters to create flexible and reusable components that can be customized for different applications.
-
Modularize your design: Break down large designs into smaller, more manageable modules to improve readability, maintainability, and reusability.
-
Document your design: Provide clear and concise documentation for your VHDL code, including a description of the functionality, input/output ports, and any assumptions or constraints.
-
Follow coding standards: Adhere to industry-standard coding guidelines, such as the IEEE VHDL Style Guide, to ensure consistency and compatibility with other designs and tools.
Frequently Asked Questions (FAQ)
- Q: What is the difference between VHDL and Verilog?
A: VHDL and Verilog are both hardware description languages used for designing digital systems. The main differences are:
- VHDL is strongly typed, while Verilog is weakly typed
- VHDL has a more verbose syntax, while Verilog has a more concise syntax
- VHDL supports multiple architectures for a single entity, while Verilog uses modules
-
VHDL is more widely used in Europe, while Verilog is more common in the United States and Asia
-
Q: Can VHDL be used for analog circuit design?
A: While VHDL is primarily used for digital circuit design, it can be used to model and simulate analog circuits using the real
data type and mathematical operations. However, specialized languages like VHDL-AMS or Verilog-A are better suited for analog and mixed-signal design.
- Q: What is the difference between a signal and a variable in VHDL?
A: Signals represent wires or connections between components and have a specific data type. They are used for communication between concurrent processes and have a default value. Variables are used for temporary storage within a process and have a local scope. They do not have a default value and are updated immediately when assigned a new value.
- Q: What is the purpose of a testbench in VHDL?
A: A testbench is a separate VHDL entity that is used to simulate and verify the functionality of a design. It instantiates the design under test (DUT), provides stimulus to its inputs, and checks the outputs against expected values. Testbenches are essential for catching errors and ensuring the design meets its specifications before synthesis and implementation.
- Q: What is the difference between synthesis and simulation in VHDL?
A: Simulation is the process of executing the VHDL code using a simulator to verify its functionality and detect any errors. It does not consider the physical implementation of the design. Synthesis, on the other hand, is the process of transforming the VHDL code into a hardware implementation, typically targeting an FPGA or ASIC. It optimizes the logic and maps it onto the available resources of the target device, generating a gate-level netlist that represents the physical implementation of the design.
In conclusion, VHDL is a powerful hardware description language that allows designers to create, simulate, and synthesize digital systems efficiently. By understanding the key concepts, language elements, and best practices of VHDL, designers can create robust, maintainable, and reusable designs that meet the ever-increasing demands of modern electronic systems.
Leave a Reply