Introduction to Attiny84
The Attiny84 is a versatile and compact 8-bit microcontroller from Microchip’s AVR family. It offers a range of features and capabilities that make it suitable for various embedded applications. In this article, we will dive into the Attiny84 Datasheet, exploring its pin configuration and programming steps to help you get started with this powerful microcontroller.
Key Features of Attiny84
- 8KB Flash Memory
- 512 Bytes EEPROM
- 512 Bytes SRAM
- 12 General Purpose I/O Pins
- 8-bit Timer/Counter with PWM
- 16-bit Timer/Counter with PWM
- Universal Serial Interface (USI)
- 10-bit ADC
- Operating Voltage: 1.8V to 5.5V
- Operating Frequency: Up to 20MHz
Attiny84 Pin Configuration
Understanding the pin configuration of the Attiny84 is crucial for designing circuits and interfacing with external components. The Attiny84 comes in a 14-pin PDIP (Plastic Dual Inline Package) or SOIC (Small Outline Integrated Circuit) package.
Attiny84 Pinout Diagram
Pin Description
Pin Number | Pin Name | Description |
---|---|---|
1 | VCC | Supply Voltage |
2 | PA0 | Port A, Pin 0 (ADC0, PCINT0) |
3 | PA1 | Port A, Pin 1 (ADC1, PCINT1) |
4 | PA2 | Port A, Pin 2 (ADC2, PCINT2) |
5 | PA3 | Port A, Pin 3 (ADC3, PCINT3) |
6 | PA4 | Port A, Pin 4 (ADC4, PCINT4) |
7 | PA5 | Port A, Pin 5 (ADC5, PCINT5) |
8 | PA6 | Port A, Pin 6 (ADC6, PCINT6) |
9 | PA7 | Port A, Pin 7 (ADC7, PCINT7) |
10 | GND | Ground |
11 | PB0 | Port B, Pin 0 (PCINT8, XTAL1, CLKI) |
12 | PB1 | Port B, Pin 1 (PCINT9, XTAL2) |
13 | PB2 | Port B, Pin 2 (PCINT10, INT0, ADC8, OC0A, CKOUT) |
14 | PB3 | Port B, Pin 3 (PCINT11, RESET, ADC9, OC1A, dW) |
The Attiny84 has two ports, Port A and Port B, each with 8 pins. These pins can be configured as inputs or outputs and have multiple functions, such as ADC (Analog-to-Digital Converter), PCINT (Pin Change Interrupt), PWM (Pulse Width Modulation), and more.
Programming the Attiny84
To program the Attiny84, you need a programmer that supports the AVR family of microcontrollers. Some popular programmers include:
- AVR ISP (In-System Programmer)
- AVRISP mkII
- USBasp
- Arduino as ISP
Setting Up the Programming Environment
- Install the Arduino IDE on your computer.
- Add support for the Attiny84 in the Arduino IDE:
- Open the Arduino IDE and go to File -> Preferences.
- In the “Additional Boards Manager URLs” field, enter the following URL:
https://raw.githubusercontent.com/damellis/attiny/ide-1.6.x-boards-manager/package_damellis_attiny_index.json
- Click “OK” to close the Preferences window.
- Go to Tools -> Board -> Boards Manager.
- Search for “attiny” and install the “ATTinyCore by David A. Mellis” package.
- Connect your programmer to the Attiny84 and your computer.
Programming Steps
- Open the Arduino IDE.
- Select the appropriate board and programmer:
- Go to Tools -> Board and select “ATtiny24/44/84”.
- Go to Tools -> Processor and select “ATtiny84”.
- Go to Tools -> Clock and select the desired clock speed.
- Go to Tools -> Programmer and select your programmer (e.g., “AVR ISP”).
- Write your Arduino sketch or load an existing one.
- Verify the sketch by clicking the “Verify” button (checkmark icon).
- Upload the sketch to the Attiny84 by clicking the “Upload” button (arrow icon).
- Wait for the programming process to complete. The Arduino IDE will display “Done uploading” when the programming is successful.
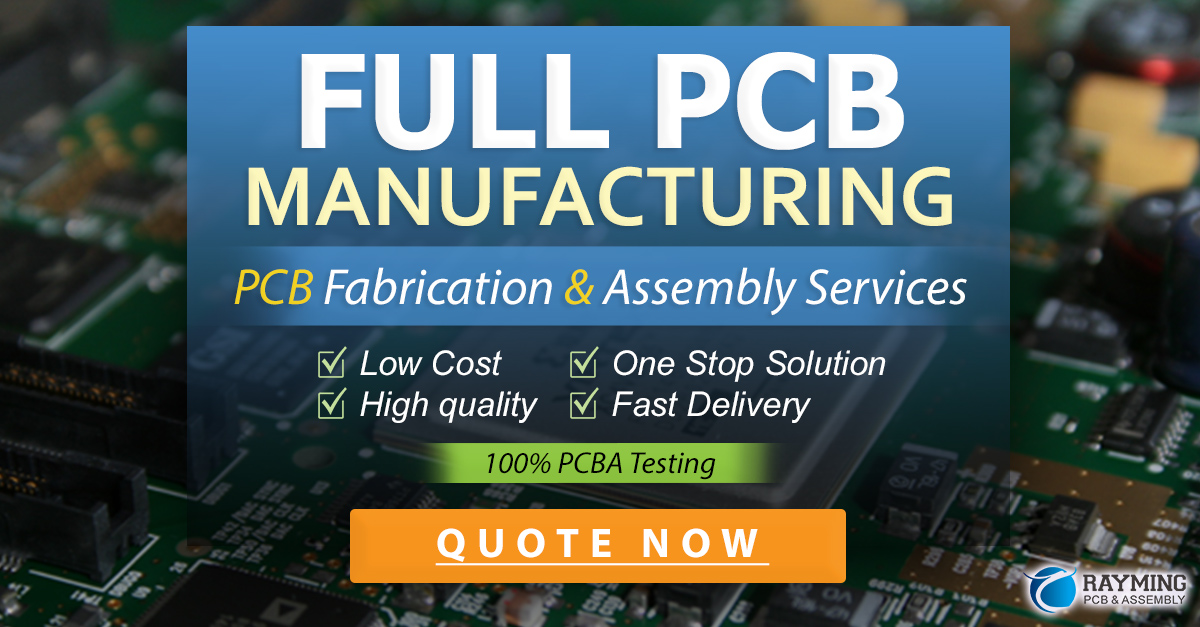
Example: Blinking an LED
Let’s look at a simple example of blinking an LED connected to the Attiny84. This example demonstrates how to configure a pin as an output and control its state.
Circuit Diagram
Code
void setup() {
pinMode(0, OUTPUT); // Set PA0 as an output
}
void loop() {
digitalWrite(0, HIGH); // Turn the LED on
delay(1000); // Wait for 1 second
digitalWrite(0, LOW); // Turn the LED off
delay(1000); // Wait for 1 second
}
In this example, we set PA0 (Pin 2) as an output using the pinMode()
function in the setup()
block. In the loop()
block, we use the digitalWrite()
function to turn the LED on and off with a delay of 1 second between each state change.
Advanced Features
The Attiny84 offers several advanced features that can be utilized in more complex projects. Let’s explore a few of these features.
Analog-to-Digital Converter (ADC)
The Attiny84 has a 10-bit ADC that allows you to measure analog signals on pins PA0 to PA7 and PB2 to PB3. To use the ADC, you need to configure the desired pin as an input and enable the ADC functionality.
Example code for reading an analog value from PA0:
void setup() {
Serial.begin(9600);
}
void loop() {
int adcValue = analogRead(A0); // Read the analog value from PA0
Serial.println(adcValue); // Print the value to the serial monitor
delay(100);
}
Pulse Width Modulation (PWM)
PWM allows you to generate analog-like signals using digital pins. The Attiny84 has two timers (Timer/Counter0 and Timer/Counter1) that can be used for PWM generation.
Example code for generating a PWM signal on PA6 (OC0A):
void setup() {
pinMode(6, OUTPUT); // Set PA6 as an output
TCCR0A = (1 << COM0A1) | (1 << WGM01) | (1 << WGM00); // Configure Timer/Counter0 for Fast PWM mode
TCCR0B = (1 << CS01); // Set prescaler to 8
OCR0A = 128; // Set PWM duty cycle to 50%
}
void loop() {
// PWM signal is generated automatically
}
Universal Serial Interface (USI)
The USI is a flexible serial interface that can be used for various communication protocols, such as SPI, I2C, and UART. It allows the Attiny84 to communicate with other devices.
Example code for using the USI as an SPI master:
#include <SPI.h>
void setup() {
SPI.begin(); // Initialize the SPI interface
}
void loop() {
SPI.beginTransaction(SPISettings(1000000, MSBFIRST, SPI_MODE0)); // Configure SPI settings
SPI.transfer(0x55); // Send data over SPI
SPI.endTransaction();
delay(1000);
}
Frequently Asked Questions (FAQ)
- What is the difference between Attiny84 and other Attiny microcontrollers?
-
The Attiny84 is part of the Attiny family of microcontrollers. It has more memory (8KB Flash, 512 bytes EEPROM, 512 bytes SRAM) compared to smaller Attiny microcontrollers like Attiny13 or Attiny25. It also has additional features such as a 16-bit timer and USI.
-
Can I program the Attiny84 using the Arduino IDE?
-
Yes, you can program the Attiny84 using the Arduino IDE by installing the ATTinyCore board package. This allows you to write and upload sketches to the Attiny84 using the familiar Arduino programming language and environment.
-
What is the maximum operating frequency of the Attiny84?
-
The Attiny84 can operate at frequencies up to 20MHz. However, the actual maximum frequency depends on the supply voltage. At lower voltages, the maximum frequency is reduced.
-
Can I use the Attiny84 for battery-powered projects?
-
Yes, the Attiny84 is well-suited for battery-powered projects due to its low power consumption. It can operate at voltages as low as 1.8V, making it compatible with various Battery Types.
-
How many PWM channels does the Attiny84 have?
- The Attiny84 has two PWM channels, one on Timer/Counter0 (OC0A on PA6) and another on Timer/Counter1 (OC1A on PB3). These channels can be used to generate PWM signals for controlling motors, dimming LEDs, or other applications requiring analog-like signals.
Conclusion
The Attiny84 is a powerful and versatile microcontroller that offers a wide range of features in a compact package. By understanding its pin configuration and programming steps, you can harness its capabilities to create diverse embedded projects. Whether you’re a beginner or an experienced developer, the Attiny84 provides a solid foundation for learning and experimenting with microcontroller programming.
Remember to refer to the Attiny84 datasheet for detailed information on its specifications, registers, and functionality. With the knowledge gained from this article and further exploration, you’ll be well-equipped to unleash the potential of the Attiny84 in your own projects.
Happy programming!
Leave a Reply